The path to reproducing a scenario for a custom is sometimes a long-ish one. I needed to include a custom assembly in an Azure Function App which I describe here “How to add assembly references to an Azure Function App” and documented this one here “Could not load file or assembly” along the way here […]
Read More →Tags: C#
Setting up and using GitHub in Visual Studio 2017
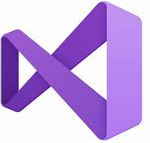
I wrote this article here about setting up GitHub in Visual Studio 2015 and felt it necessary to document the same in Visual Studio 2017. Here is how I setup GitHub source code management in Visual Studio 2017 Community. Install the GitHub extension for Visual Studio Create your GitHub repo and then login Create a […]
Read More →How to enable C# 7 in Visual Studio 2017
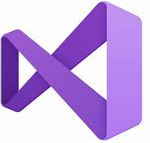
I was working with Visual Studio 2017 and found that 4.6.2 is not installed as default yet, so I wrote this article here about that. In that article I also point out that you need to install the System.ValueType NuGet package to the Tuples capabilities to work. This time I wanted to get the Pattern […]
Read More →Throwing and catching exceptions using try catch in C#
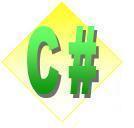
Catching exceptions within your code make a huge difference in how your program will be perceived from a user. If you do not catch the exception then in many cases your program will crash and be required to close and then reopened. However, if you catch exceptions you can handle them gracefully. You gracefully recover […]
Read More →How to create and search a Lucene.Net index in 4 simple steps using C#, Step 4
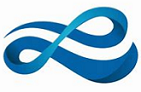
Display the results. To view the results we need to loop through the ScoreDoc array we created in Part3. We do this by iterating through it within a for loop. for (int i = 0; i < hits.Length; i++) { int docId = hits[i].doc; float score = hits[i].score; Lucene.Net.Documents.Document doc = searcher.Doc(docId); string result = […]
Read More →How to call an async method from a console app main method
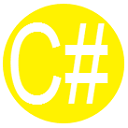
I find myself needing to look this code snippet up time to time so I thought I’d write it up. Check out some of my other articles I wrote in regards to ASP.NET Core and .Net Core How to call an async method from a console app main method How to deploy a .NET Core […]
Read More →Source Code plug in for WordPress blogs using Open Live Writer
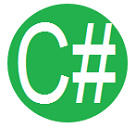
I like to write. I use WordPress at the moment for my blogs, I think as the blogs are stored in a database instead of being static text when I want to move to another platform or technology, I will only need to export the data and format it to match the new platform requirements. […]
Read More →LINQ to XML vs. XML DOM, XMLDOM and LINQ to XML with Lambda Expression
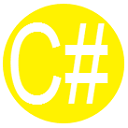
There a numerous reasons why you would need to create an XML file. Configuration settings or using it as a data source are 2 examples. In both cases, you would need to create the XML file. If you have never done it before then the first question is, How do I create, read and modify […]
Read More →Create and deploy an ASP.NET Core Web API to Azure Windows
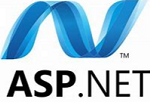
There are a number of things I want to accomplish with this and a few future articles: How to deploy an ASP.NET Core Web API to an Azure App Services Web App How to deploy an ASP.NET Core Web API to an Azure VM Check out some of my other articles I wrote in regards […]
Read More →Using LINQ to Reflection with Lambda Expressions in C#
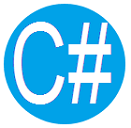
I think Lambda expression are cool. Not only are cool, they are pretty powerful and can make your code a lot more readable and compact. I wrote this article here about how to simply use LINQ to Reflection to list the methods and types of an assembly. In this example I will use the same […]
Read More →