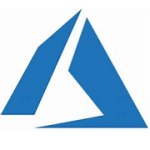
I was reading this article where it discusses some of the different kinds of Clouds that Azure supports. For example: Public Cloud China Cloud German Cloud US Government Cloud There may be more, but I was working on publishing an Azure App Service to the German Cloud and I was not finding my subscription in […]
Read More →