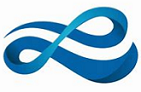
I used this code to perform a search of a Lucene.Net index. Lucene.Net.Store..Directory directory = Lucene.Net.Store.FSDirectory .Open(new DirectoryInfo(textBoxSearchIndex.Text)); Lucene.Net.Analysis.Analyzer analyzer = new Lucene.Net.Analysis.Standard .StandardAnalyzer(LuceneUtil.Version.LUCENE_29); Lucene.Net.Search.Searcher searcher = new Lucene.Net.Search .IndexSearcher(LuceneIndex.IndexReader .Open(directory, true)); Lucene.Net.Search.Query query = new Lucene.Net.QueryParsers .QueryParser(LuceneUtil.Version.LUCENE_29, “contents”, analyzer) .Parse(textBoxSearch.Text); Lucene.Net.Search.Hits hits = […]
Read More →