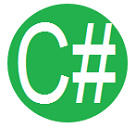
I wrote previously a post where I combine 2 Lists together here. Recently, I had the requirement to join 2 dictionaries together. I needed to build a DataTable and an NHibernate HQL query dynamically where the column headings could be different than the name of the actual database column name. To do this I created […]
Read More →