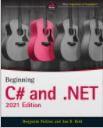
I would like to proudly announce the release and availability of my new Beginning C# and .NET 2021 Edition book, which was co-authored with Jon Reid. The book contains over 800 pages of material relating to the skills and knowledge required to become a great C# programmer. The book is designed around the premise that […]
Read More →